Convert Timestamp to Date in PHP: A Comprehensive Guide
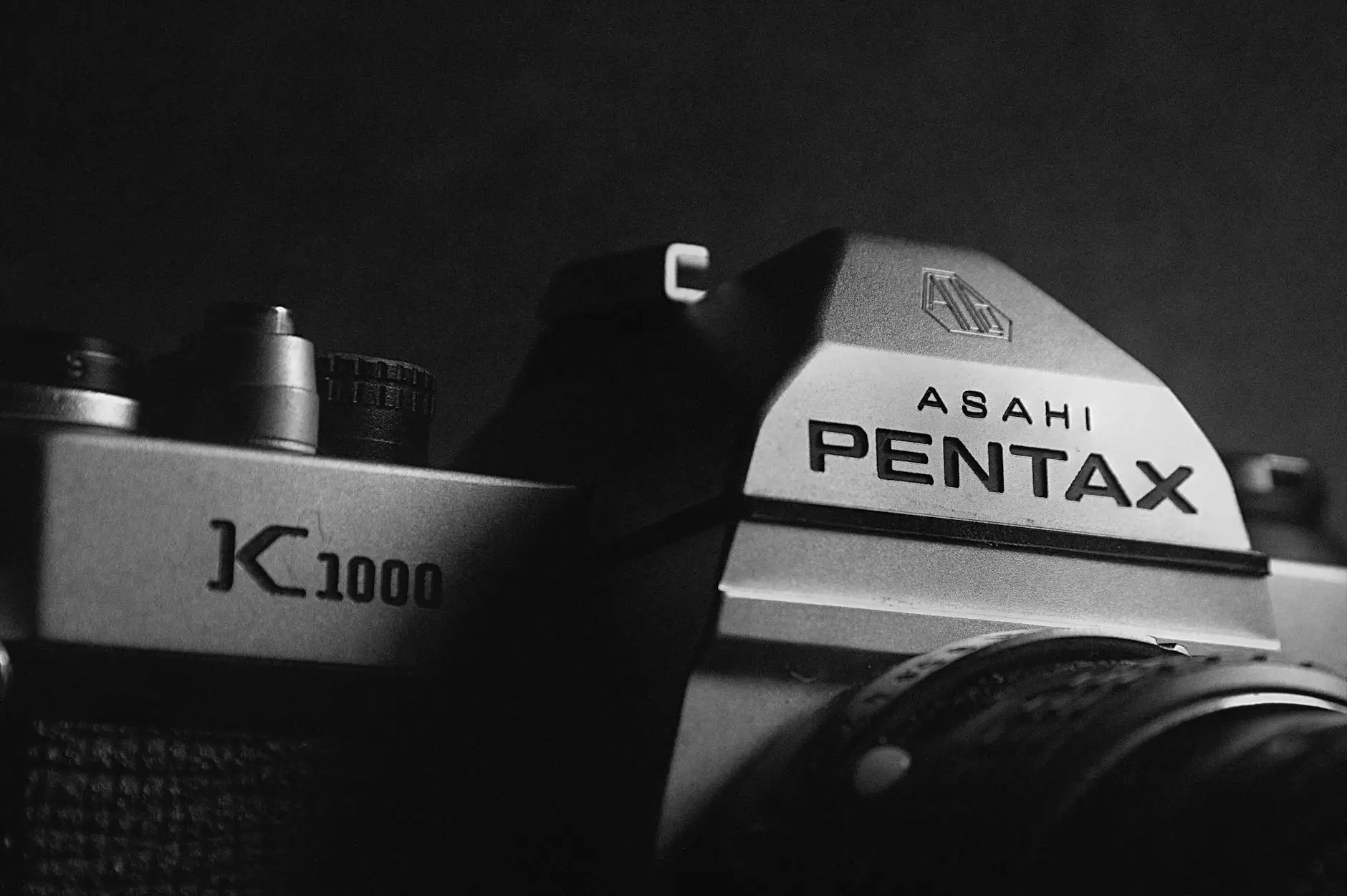
In the world of web development, managing dates and times can often be a complex endeavor, particularly when dealing with timestamps. Timestamps, represented as the number of seconds since the Unix Epoch (January 1, 1970), are frequently used in programming for their simplicity and efficiency. This article aims to delve into how to convert timestamp to date in PHP while exploring various techniques and best practices.
Understanding Timestamps
A timestamp is a crucial concept within programming and database management. It serves as a numerical representation of a specific point in time, which is convenient for sorting and storing date values. In PHP, timestamps can be generated using the time() function, which returns the current Unix timestamp, or by utilizing strtotime() to convert date strings into timestamps.
Why Use Timestamps?
Timestamps offer several advantages:
- Consistency: Timestamps provide a uniform point of reference across different time zones and locations.
- Database Efficiency: Storing dates as integers can lead to faster queries and operations in databases.
- Easy Calculation: Performing calculations on timestamps is straightforward, allowing developers to easily calculate durations, add or subtract time, and convert formats.
Converting Timestamp to Date in PHP
The PHP programming language simplifies the process of converting timestamps into human-readable date formats through its built-in functions. The most commonly used function for this purpose is date() combined with a proper format string.
Using the date() Function
The date() function in PHP interprets a given timestamp and formats it according to the specified parameters. The syntax is as follows:
date(format, timestamp)Here, format is a string representing the desired output format, while timestamp is the Unix timestamp you want to convert. If you omit the timestamp argument, date() defaults to the current time.
Example 1: Basic Conversion
Consider the following example that illustrates how to convert a timestamp into a formatted date:
Understanding Format Strings
The format string you use with the date() function is crucial as it determines the output. Some common format characters include:
- Y - Full numeric representation of a year (4 digits)
- m - Numeric representation of a month (01 to 12)
- d - Day of the month (01 to 31)
- H - 24-hour format of an hour (00 to 23)
- i - Minutes (00 to 59)
- s - Seconds (00 to 59)
Example 2: Custom Date Formatting
To customize our output further, we can modify our format string:
Handling Time Zones
When working with timestamps, it's essential to consider time zones. By default, PHP uses the server's time zone settings, which might not always align with your application requirements.
Setting the Time Zone
Use the date_default_timezone_set() function to specify a time zone:
Common Use Cases
Understanding how to convert timestamp to date in PHP is beneficial in various scenarios:
1. Displaying Dates in User Interfaces
Often, you need to display dates in a user-friendly format on web applications and interfaces. By using the date() function, you can easily format and present timestamps for display.
2. Sorting and Filtering Data
When dealing with data that includes timestamps, such as logs or events, it's imperative to convert them into readable dates for sorting or filtering purposes. Correctly formatted dates provide better clarity and ease of analysis.
3. Generating Reports
Reports often require dates formatted in particular styles. You can convert timestamps to the required formats using PHP functions, making data presentation seamless.
Advanced Date Operations in PHP
PHP offers several advanced techniques for date manipulation beyond mere conversion of timestamps. For example, the DateTime class allows for more structured date-time handling.
Using the DateTime Class
The DateTime class provides a robust interface to work with date and time. It can be used to create date objects from timestamps:
Benefits of Using DateTime
- Object-Oriented:
- Supports time zone management effectively.
- Allows for comprehensive date manipulation, such as adding or subtracting intervals.
Example of Date Manipulation with DateTime
Here’s a quick example of adding a month to a given timestamp:
Conclusion
In summary, mastering how to convert timestamp to date in PHP is vital for any web developer. By utilizing the date() function and DateTime class, you can effectively work with date and time in your applications. This knowledge not only enhances your coding capabilities but also improves the overall user experience of your web applications.
Whether you are displaying dates, generating reports, or handling user input, understanding time representation in PHP is a critical skill. By leveraging the tools at your disposal, you can ensure that your applications remain both functional and user-friendly.
Explore More with Semalt.Tools
If you want to dive deeper into web design and software development, visit semalt.tools. We offer comprehensive resources and tools to help you grow your skills and enhance your projects.
convert timestamp to date php